how to check if char is digit in python: A Simple Guide
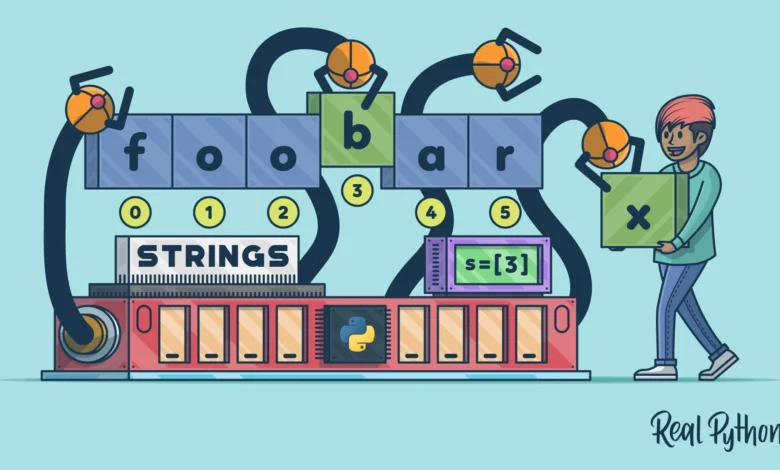
When you’re working with Python, you might want to know how to check if a character is a digit. If you’re wondering how to check if char is digit in Python, you’re in the right place! This is a simple task that can be done in a few easy steps. In this post, we’ll walk you through the process and explain it in a way that’s easy to understand.
Checking if a character is a digit means you’re testing whether the character is a number. In Python, there are built-in methods to help you with this, making your job much easier. Let’s dive in and learn how to check if char is digit in Python with a simple example!
Understanding the Basics: How to Check if Char is Digit in Python
When you’re working with Python, you may often need to check if a character is a digit. This is useful when you want to make sure the input you’re working with is a number. So, how do you check if a char is a digit in Python?
Python makes it easy with built-in functions. The most commonly used method is isdigit(). This method checks whether the character is a numeric digit. If the character is a digit, it will return True; otherwise, it returns False. This is a great tool to use when working with strings or user input.
Step-by-Step Guide: How to Check if Char is Digit in Python
To check if a character is a digit in Python, you first need to have a string or character you want to test. You can then use the isdigit() method on that character. Here’s how you can do it step by step:
- Take the input string or character.
- Apply the isdigit() method on the character.
- The method will return True if it’s a digit, and False if it’s not.
This simple code will help you check if the character is a digit or not. The isdigit() method is a very useful tool for tasks like this, making your coding easier and faster.
Why Checking if Char is Digit in Python Matters in Programming
When you’re building a program, it’s important to make sure that the data you’re working with is in the right format. For example, when you need to handle user input, checking if the character is a digit helps you avoid errors later on.
If the input is expected to be a number, you can ensure that it is a valid digit before doing any calculations or comparisons. This can prevent mistakes and make your code more reliable. In real-world scenarios, you will often be working with user input or processing data, and checking for digits is a common task.
Common Mistakes When Trying to Check if Char is Digit in Python
When learning how to check if char is digit in Python, beginners often make some common mistakes. Let’s look at a few of them to help you avoid these pitfalls:
Mistake 1: Using isdigit() on Non-String Types
- The isdigit() method only works on strings. Trying to use it on a number or other data types will cause an error. Always make sure you’re using it on a string.
Mistake 2: Confusing Digits with Numbers
- The isdigit() method checks for characters that are numeric digits (0-9). It does not work for decimal numbers, negative signs, or other types of numeric values like floats.
Conclusion
In this post, we’ve learned how to check if char is digit in Python. By using Python’s isdigit() method, checking if a character is a digit is easy and quick. Whether you’re a beginner or more experienced with Python, this simple method can help you ensure that your data is in the right format before you move forward with your code.
So, next time you need to check if a character is a digit, just remember to use isdigit(). It saves time and prevents errors, making your Python coding smoother and more reliable. Keep practicing, and you’ll get even better at using these simple but powerful Python tools!
FAQs
Q: How do I check if a character is a digit in Python?
A: You can use the isdigit() method in Python. It checks if a character or string is a digit and returns True if it is, and False if it’s not.
Q: Does isdigit() work on spaces?
A: No, the isdigit() method does not consider spaces as digits. It will return False for a space or any special characters.
Q: Can I use isdigit() on numbers in Python?
A: No, isdigit() works on strings. If you want to check if a number is a digit, you should first convert it to a string.
Q: Will isdigit() return True for decimal numbers?
A: No, isdigit() only checks for digits from 0-9. It does not work for decimal numbers or any characters like a period or minus sign.