Troubleshooting the Godot is_pixel_opaque not working
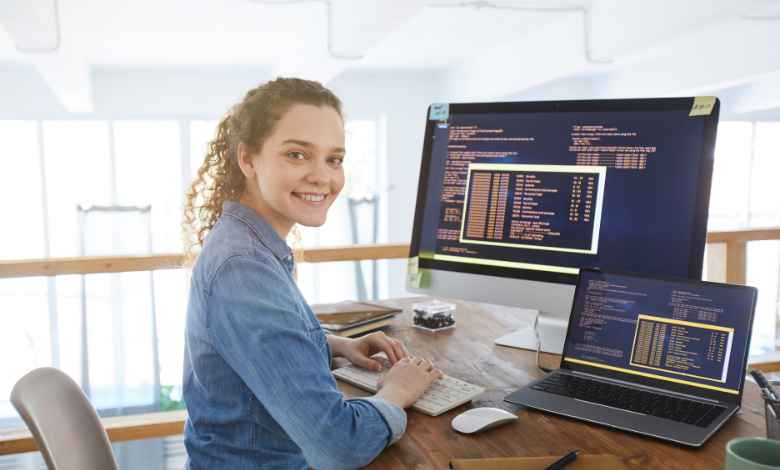
Godot Engine is a versatile and popular game development platform used by both beginners and professionals alike. One of its useful functions is is_pixel_opaque()
, which helps check the transparency or opacity of specific pixels in a texture or image. However, some developers encounter issues where is_pixel_opaque()
does not work as expected. This article will explore the function in detail, common problems developers face, and possible solutions to get it working correctly.
What is is_pixel_opaque()
?
Before delving into the troubleshooting, let’s clarify what theis_pixel_opaque
()
function does in Godot. This function determines if a specific pixel in an image is fully opaque, meaning it checks whether the alpha channel of the pixel has a value of 255 (completely opaque). This is useful in various situations, such as collision detection with transparent textures, selecting objects, or implementing custom rendering effects.
The function is typically used as follows:
var image = load("res://path_to_image.png").get_image()
var is_opaque = image.is_pixel_opaque(x, y)
Here, the function checks if the pixel at coordinates (x, y)
in the loaded image is opaque or not. The return value is true
if the pixel is opaque, and false
if it’s partially or fully transparent.
Common Reasons Why is_pixel_opaque()
Might Not Work
While the function is relatively straightforward, there are several reasons why is_pixel_opaque()
may not work as expected. Let’s look at the most common issues and how to resolve them.
1. The Image Format Lacks an Alpha Channel
One of the primary reasons is_pixel_opaque()
fails is that the image being used does not contain an alpha channel. If the image format is RGB instead of RGBA, the function won’t properly determine opacity because there is no transparency data.
Solution: Ensure that the image you are working with has an alpha channel (RGBA format). You can check and convert the image format in your graphic editing software, such as GIMP or Photoshop, to ensure that transparency information is available.
Alternatively, you can also check if the image has an alpha channel in Godot by inspecting the image resource and ensuring that it’s using a format like Image.FORMAT_RGBA8
.
2. The Coordinates Are Out of Bounds
Another common issue arises when the coordinates passed to the function are outside the bounds of the image. In such cases, the function may return unexpected results or crash.
Solution: Make sure the (x, y)
coordinates you pass to is_pixel_opaque()
are within the image dimensions. You can do this by using the get_width()
and get_height()
methods of the image to verify:
var image = load("res://path_to_image.png").get_image()
if x >= 0 and x < image.get_width() and y >= 0 and y < image.get_height():
var is_opaque = image.is_pixel_opaque(x, y)
else:
print("Coordinates are out of bounds!")
This ensures that you only query pixels within the image’s boundaries.
3. Compression and Filtering Issues
If your images are compressed or have certain filters applied, such as mipmaps, this can affect the way pixels are read, which might lead to is_pixel_opaque()
not working as expected.
Solution: Ensure that the images you are loading are not compressed in a way that would affect pixel-level checks. You can disable compression or mipmaps for the image in the import settings:
- Go to the Godot editor and select the image file.
- In the Import tab, set Compression to Lossless or Uncompressed.
- Uncheck Mipmaps if enabled, as they can affect pixel precision at different levels of detail.
- Reimport the image after applying these settings.
4. Incorrect Use of get_image()
The is_pixel_opaque()
function is applied to an Image
object, but sometimes developers mistakenly use it directly on a Texture
object. Since Texture
objects are not directly compatible with this method, you must extract the underlying image using get_image()
before using is_pixel_opaque()
.
Solution: Make sure you are using get_image()
to retrieve the actual Image
object from the texture, as shown below:
var texture = load("res://path_to_texture.png")
var image = texture.get_image() # This is crucial for is_pixel_opaque to work!
var is_opaque = image.is_pixel_opaque(x, y)
5. Alpha Values Not Set Correctly
Sometimes, images may appear transparent in parts, but the alpha values in those regions are not fully set to zero. As a result, is_pixel_opaque()
may return true
even though the region looks transparent due to partial transparency or shading.
Solution: Use an image editor to inspect the alpha channel of your image to ensure that fully transparent areas have an alpha value of 0. This will prevent false positives when using is_pixel_opaque()
.
6. Godot Version Bugs
As with any development tool, specific versions of Godot may have bugs or issues with certain functions. It’s possible that in some versions of Godot, is_pixel_opaque()
might not behave as expected due to an internal engine issue.
Solution: Make sure you are using the latest stable version of Godot. Check the Godot GitHub repository or the official release notes to see if any known bugs related to is_pixel_opaque()
have been addressed in recent versions. If you are experiencing issues in a specific version, consider upgrading or downgrading to a different version.
7. Problems with Atlas Textures or Packed Textures
If you’re using textures that are packed into an atlas, the coordinates may be incorrect when querying a specific pixel. Similarly, when working with sprite sheets or packed textures, the function might not behave as expected.
Solution: If you’re working with an atlas, ensure that the coordinates you’re passing to is_pixel_opaque()
are local to the image and not the entire texture atlas. You may need to manually adjust the coordinates based on the region used for that specific sprite or texture.
Final Thoughts
The is_pixel_opaque()
function in Godot can be an invaluable tool when working with images and textures, but several common issues can lead to unexpected behavior. By ensuring your image formats are correct, validating the coordinates, and checking for issues with compression or Godot versions, you can get the function to work reliably. Following these troubleshooting steps will help you avoid the most common pitfalls and use is_pixel_opaque()
effectively in your projects.
If you still encounter problems after trying these solutions, consider reaching out to the Godot community via forums or GitHub to seek further assistance.